Being an open-source programming language, the basic purpose of JavaScript is to create web-centric applications. This language is lightweight and interpreted, making it a lot more faster when compared to other languages. Apart from this, JavaScript can also be used for email validation.
If you are interested in learning email validation in JavaScript, this article is going to be more than helpful. So, let’s start without any further ado!
What is Validation?
Validation is a process for checking a user's input values. It plays a critical role in designing web applications and improves the overall user experience. This process allows us to validate mobile numbers, emails, passwords, and numerous other fields.
Some Words about Email Validation

Email validation is a viable way to determine whether an email is valid or not. If a particular email address isn’t valid or real, it can't be used for an email marketing campaign. On the contrary, valid email addresses are real and are currently used by individuals or companies.
Email validation is crucial for keeping the bounce rate to a minimum and boosting conversions. Here are a few practical applications for email validation in JavaScript:
- Landing page registration forms
- Account Registration
- Newsletter signups
- eCommerce transactions
Implementing a simple JavaScript email verification code on any of the above-mentioned pages can make a huge difference.
Why Validate Emails with JavaScript?
JavaScript is a great option when it comes to validating an email address on the client side. This speeds up the validation process, as it ensures faster processing of data. JavaScript validation is relatively more efficient as compared with server-side validation.
Listed below are the most noteworthy reasons to use JavaScript for email validation.
- JavaScript is easy to use and requires less effort to implement.
- With its agile nature, you can swiftly validate emails.
- When using JavaScript, you are putting less strain or load on a webpage.
- You won’t experience negative impacts like slowdown or increased load times.
- JavaScript is a prevalent language, which renders it highly compatible with a variety of platforms or websites.
Understanding Email Format
By knowing the right email format, you can distinguish between valid and invalid email addresses. An email address is referred to as invalid if it uses improper syntax. Without a proper format for email validation, a visitor can continue with the registration form by simply adding any sequence of characters. Hence, it is highly recommended that you rely on a proper email format.
Structure of a Valid Email Address
When it comes to the structure of a valid email address, it always comprises two main components, including ‘Username’ and ‘Email Domain Name.’
For instance, the email address ‘smith.root@yahoo.com’ is valid. This is because it contains a username (smith.root) and a domain name (company.com). Both these parts of an email address should be separated by the @ symbol.
Below is another example of a valid email address created with proper syntax:
reversecontact@gmail.com.
In this particular example, ‘reversecontact’ is the username, and ‘gmail.com’ is the entire domain name.
How to Validate Email Using JavaScript?
Email validation is an essential part of any HTML form validation. A valid email address is a subset or string of ASCII characters separated by the @ symbol. The first part refers to personal or company information, and the second part relates to the domain name.
The first part usually contains the following ASCII characters:
- Lowercase and Uppercase letters (A-Z and a-z)
- Special Characters - ! # % $ & ' * = - + / ? ^ `_ { } |~
- Numeric Characters (0 to 9)
- Period, dot, or full stop (.) can be used but not consecutively. Besides, period can’t be used at the start or end of an email address.
The domain name might contain different characters, including Letters, Digits, Hyphens, and Dots. Using regular expressions is one of the popular ways to validate email in JavaScript. It relies on regular expressions to describe or define a pattern of characters.
Using email.js File
This file offers JavaScript code, which is required to validate an email address. For email validation on the client side of a web application, the use of regular expressions is quite common.
function ValidateEmail(input) {
var validRegex = /^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9-]+(?:\.[a-zA-Z0-9-]+)*$/;
if (input.value.match(validRegex)) {
alert("Valid email address!");
document.form1.text1.focus();
return true;
} else {
alert("Invalid email address!");
document.form1.text1.focus();
return false;
}
}
Define a regular expression for validating email address
Check if the input value matches the regular expression
If it does match, send an alert stating “valid email address.”
If it doesn’t match, send an alert stating “invalid email address.”
Using email.html File
This file offers the base HTML code with defined elements for an email validation form.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>JavaScript Email Validation</title>
<link rel="stylesheet" href="email.css" type="text/css" />
</head>
<body onload="document.form1.text1.focus()">
<div class="mail">
<h2>Input an email address</h2>
<form name="form1" action="#">
<ul>
<li><input type="text" name="text1" /></li>
<li> </li>
<li class="validate">
<input
type="submit"
name="validate"
value="Validate"
onclick="ValidateEmail(document.form1.text1)"
/>
</li>
<li> </li>
</ul>
</form>
</div>
<script src="email.js"></script>
</body>
</html>
For some characters, there are certain rules. For instance, periods are normally used in an email address (i.e., smith.bob@domain.com), but they shouldn’t be used at the start or end of the username.
In addition, email service providers don't accept all characters. You might create using a valid email with a particular email provider but might face an issue while using it on another email service provider that doesn’t allow special characters to be used in your email.
If applicable, email addresses could also permit subdomains. In these circumstances, you can differentiate a subdomain from the main domain by adding a period between these two. Example include:
“john@marketing.company.com”.
Importance of Correct Email Format
An invalid email address listed in your contact data isn’t going to do any benefit, as it will lead to a bounce. A high email bounce rate could drastically impact the overall performance of your email marketing campaign.
In addition, it will also lower the sender's score or, in other words, damage the sender's reputation. This particular score is issued by the Internet service providers (ISPs) on a 100-point scale. Obviously, 100 is the best score, and 0 is a nightmare for any marketer.
Why Does Sender Reputation Matter?
The sender score reflects the email-sending behavior of a company. The following are the attributes, which can lower your sender score:
- High email bounce rate
- Poor open and click rates
- High spam complaint rate
This suggests an email address with a low sender score can’t deliver a message to the assigned address. A low score explains that ISPs distrust your email content or they question your legitimacy. As a result, the emails sent by such an address will land in the spam folder.
What is a Regular Expression?
It’s a string of text used to identify and match the patterns present in that string.
Described below is an example of regex, which is normally used for email address validation:
^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,6}$
It’s possible to use or incorporate this regex into basic JavaScript for email validation:
Javascript
function validateEmail(email) {
const pattern = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,6}$/;
return pattern.test(email);
}
// Example usage:
const email = 'test@example.com';
if (validateEmail(email)) {
console.log('Valid Email Address');
} else {
console.log('Invalid Email Address');
Alternative Basic Email Validation Methods Using JavaScript
The above-mentioned front-end email validation method using JavaScript is just a pattern check. Below, we have listed some of the most advanced email validation methods:
For this purpose, you can use a pre-existing JavaScript library
These libraries come with a more complex series of checks and patterns to validate email addresses. With this script, you can detect a vast number of variables, such as character type or whether these characters match a predefined pattern.
Examples:
Contains(str, seed [, options])
check if the string contains the seed.
options is an object that defaults to { ignoreCase: false, minOccurrences: 1 }.
Options:
ignoreCase: Ignore case when making a comparison, default false.
minOccurences: Minimum number of occurrences for the seed in the string. Defaults to 1.
isFullWidth(str)
check if the string contains any full-width chars.
isLowercase(str)
check if the string is lowercase.
isNumeric(str [, options])
Check if the string contains only numbers.
isWhitelisted(str, chars)
Check if the string only consists of characters that appear in the whitelist chars.
isEmail(str [, options])
Check if the string is an email.
With the help of these validators, you can consider different variables. For instance, you can work with variables like checking for numbers, identifying the casing of different characters, or allowing/disallowing based on a blacklist or whitelist.
You can also find a full list of validators (advanced) and options by using a repository like Github or simply downloading the file from the links provided above.
The JavaScript libraries for Email validation offer all these features. You can also take advantage of the unique validation error message or error code for creating the syntax and patterns without any hassle.
Mailbox Pinging or SMTP Check
Simple Mail Transfer Protocol (SMTP) is a primary method used by the internet for receiving and sending emails.
SMTP starts with the sending server, which sends an initial request. This is also referred to as EHLO (Extended Hello) command. Once the identity of the receiving server is confirmed by the sender, the process of emailing continues.
By leveraging this SMTP system, we can also validate an email address. You can contact the domain mentioned in the email and confirm whether the email domain exists or is in use. For this purpose, you need to connect via an API with the external email validation service.
DNS lookups
Even with an existing email domain and proper syntax, an email address won’t be considered valid. To receive incoming mail, the DNS entries of a domain must have the Mail Exchange (MX) record. By performing a DNS lookup, you can verify whether an email address exists or is usable.
Best Practices for JavaScript Email Validation
Cleaning up the incoming email addresses with JavaScript isn’t just essential for the performance of your campaign, it’s also vital to prevent harmful or spammy data.
Here are the best practices for better security of our platform or site when performing email validation.
Apply JavaScript Email Validation to a Basic HTML Form
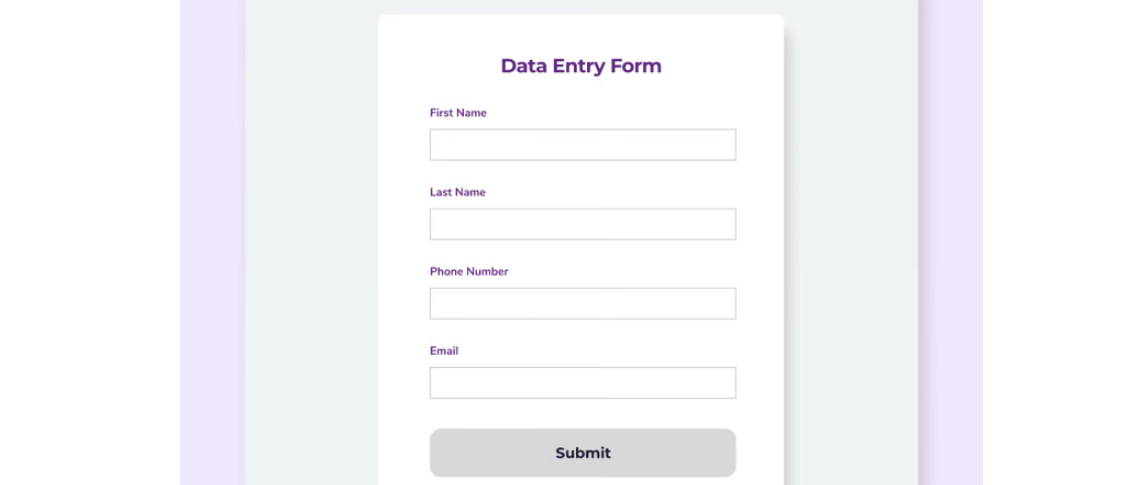
For any platform or web page containing a data entry form, make sure to use JavaScript email verification. Although it’s a basic form, the script will help you detect malformed entries and block out the data, which isn’t suitable for the field.
By doing so, you can block invalid information and offer a better user experience by providing instant error feedback to the user. In most circumstances, visitors usually make mistakes while entering the data. It allows them to understand what went wrong.
Take Advantage of Sanitizers from JavaScript Libraries
When you are using a library such as validator.js, you can access the sanitizers. These offer a wide range of ways to replace or eliminate unwanted characters from a provided entry. A few examples include:
Blacklist: This list allows you to block specific characters. For instance, if any one of the listed characters appears in the data field, it is automatically removed.
Whitelist: It’s the opposite of a blacklist, which helps you to create a list of characters that are allowed. Any other character that isn’t on this list would be automatically blocked.
NormalizeEmail: This feature automatically updates an entry and makes sure it complies with the rules set by various mail providers. For instance, you can consider using rules about case sensitivity. Sites not relying on any kind of character entry sanitization are prone to attacks.
Hackers are well aware of the fact that how they can manipulate the vulnerabilities. They can easily enter strings, which can allow unauthorized access to your platform. This risks the personal information on your platform.
Use Clear Error Messages
While it is essential to block harmful entries, you should also consider telling your users why the error occurs. This information will give a hint to the users about the mistake that they have just made. As a result, users can correct the mistake and continue with the process. This is crucial, as your goal is to retrieve valid information from your users.
Retest the Emails with Past Validation
Even if the email address typed by a user matches a predefined regex, there’s a guarantee that it is valid. For instance, a misspelled entry could also be considered as an appropriate syntax.
For example, Peter wants to write his email address “peter@gmail.com,” but instead submits “petor@gmail.com.” This will be considered a valid email address according to your JavaScript email pattern check.
This is possible as JavaScript fails to perform more advanced techniques like DNS lookup or SMTP check. Although you can send an email to this address, it will bounce due to being treated as invalid. As a result, the bounce rate will increase, causing problems including a lower email deliverability rate.
JavaScript Limitations for Email Validation
Generally, JavaScript works well most of the time as far as email validation is concerned. However, there are certain limitations linked with this particular method including:
- A regex pattern only checks for the allowed/disallowed characters. It fails to verify the email domain.
- DNS or SMTP checks tend to be resource-intensive, which isn’t viable for most of the servers.
- Performing DNS lookups or SMTP checks isn’t possible by merely using JavaScript.
The first limitation presents a major issue, as the email address passed the check might not be valid or one that is in use. Hence, you have no other way but to use domain verification and Simple Mail Transfer Protocol (SMTP checks).
An email validation tool should be able to verify the domain and check its authenticity. This is essential, as in some cases, the destination exists, but you are unable to send a message. However, JavaScript won’t validate email addresses to such an extent.
Even if you are capable of using advanced email validation procedures along with JavaScript, the whole process would be a lot more demanding for the everyday servers. Besides, things can be more challenging if you are experiencing high traffic volume and need bulk validations.
Final Thoughts
Once you have gone through the above guide, it will be a lot easier for you to do email validation in JavaScript. The topic might seem to be difficult, but it isn’t the case. With some basic knowledge of JavaScript, you can easily perform the task of email validation using JavaScript. By relying on the best and most effective email validation techniques, you can avoid using invalid email addresses and reduce bounce rates.